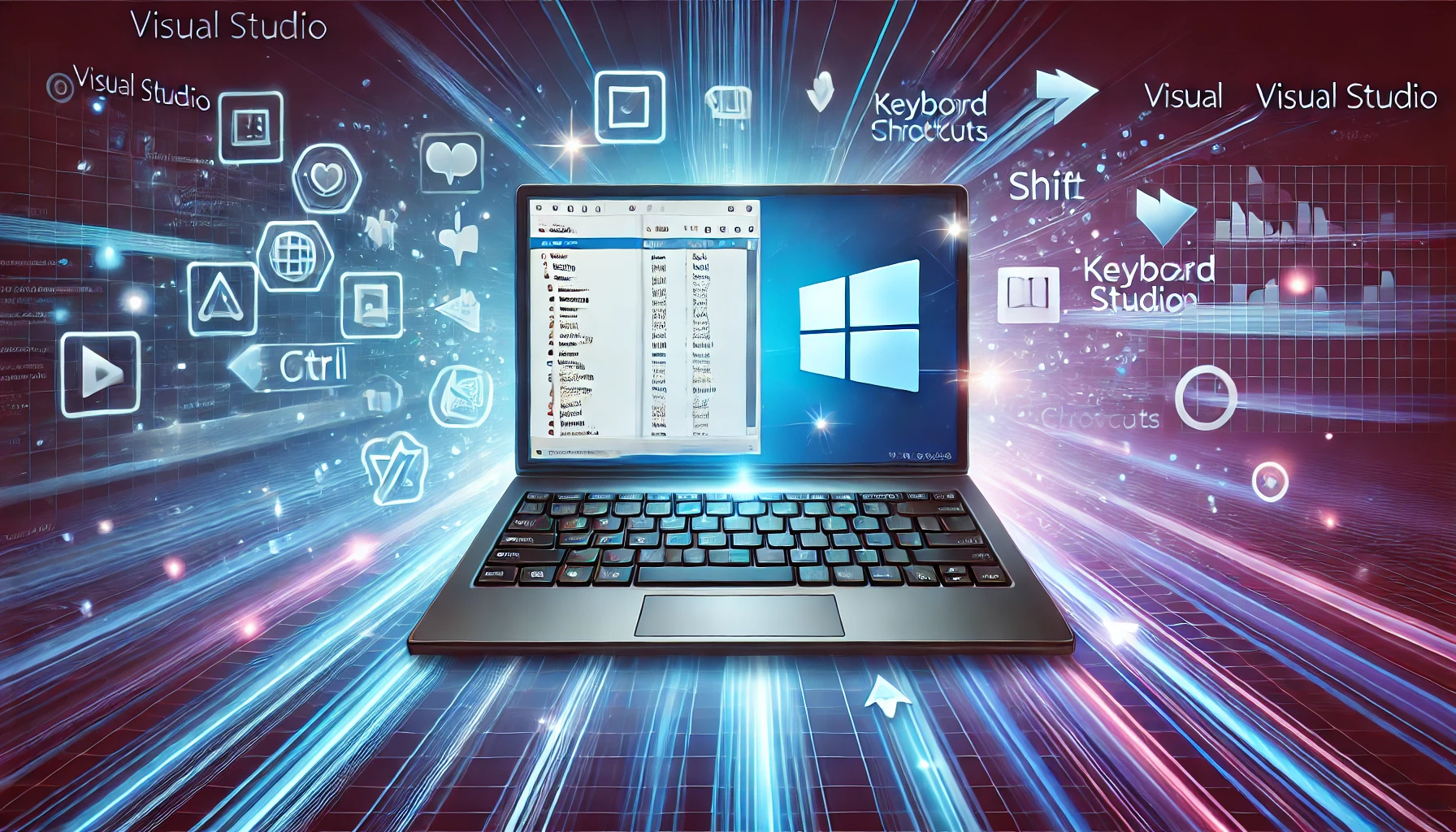
Visual Studio Tips & Tricks — Shortcuts
The next part of this series contains various tips to get code on the page faster. These are shortcuts built into Visual Studio to make your life easier, taking some of the monotony away from the more mundane aspects of programming.
Code Snippets
Starting off with the ultimate time-saving tip, code snippets are pre-made pieces of code that can be generated using as little as two letters. These snippets come up in IntelliSense when typing, however, it isn’t instantly clear what they are or how to use them. For this example, I’ll be showing the “prop” snippet which will generate a property with a getter and setter.
First type “prop” into the editor:
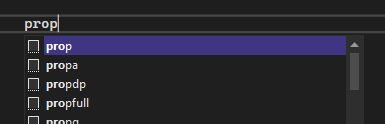
Next, hit Tab twice. The basic code snippet will appear:
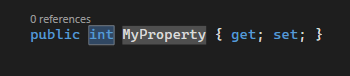
Currently, the “int” type is highlighted. If you are declaring an int then move on to the next step, otherwise, change this to the desired type by typing it in (no need to click). I will change mine to a string.
Once you have entered the type, press Tab twice again:

Now the property name is highlighted. Type in the desired property name and press Enter.

Your property is now ready to use.
It is also possible to create your own snippets if there is code that you write often.
A full list of default snippets is available on the Microsoft Docs site.
Note: I have personally found “sw” to be invaluable when needing to generate a switch statement from an enum.
Intellisense
Possibly the most common feature of an IDE is the ability to suggest code based on what you are typing via a code completion tool. Visual Studio’s Intellisense is great at what it does, but there are a few features that aren’t as well known.
First up is the ability to complete based on a camel case. If you have a method named “GetAllProducts()” then simply type in “gap” and it should find it.
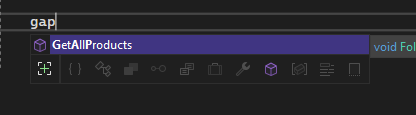
Next are the filters. If you have a long list and aren’t certain of the name of what you are looking for, but you do know the type, then the filters located at the bottom of the IntelliSense window can be used to narrow down the list. Hovering over each one will tell you what it does.
It’s not something I use that often, but it is handy to know it is there.
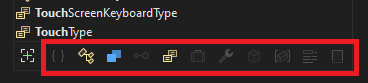
The last is one that I only started using recently but has saved me a tonne of time. Clicking the + icon or pressing Alt + A with the IntelliSense window open will enable lookup in unimported namespaces. Normally, IntelliSense will be limited to the namespaces from the usings at the start of the file.
Great if that is what you need, not so great if you want to reference anything outside of it. Enabling this option will allow you to search your entire codebase and automatically add any missing using statements.
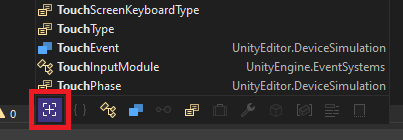
Intellicode
This one has been enabled in Visual Studio since Visual Studio 2019 16.4, so if you keep your IDE up to date then you will most likely have seen Intellicode suggestions.
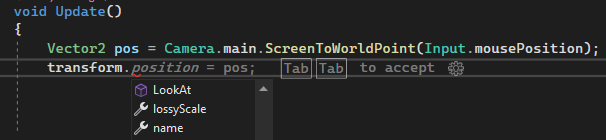
Utilising AI, Intellicode will use the developer’s “current code context and patterns” to predict the suggestion, rather than just an alphabetical list. It explains how to use it in the line itself, so this is more of an FYI.
My experience with Intellicode suggestions has been pretty mixed. It has provided me with absolute gems that saved me looking up code in other places while also giving me some incredibly unhelpful results. I would rather have it than not, as there is no downside to simply ignoring the suggestion.
Complete Word (Ctrl + Space)
Another incredibly useful command that will autocomplete the word you are typing once IntelliSense has whittled down the list of possibilities to one. Not that useful for short names, but amazing for ThatOneIncrediblyPreciseMethodThatHasToBeThisLongForSomeReason().
To use it, simply start typing and press Ctrl + Space, if nothing happens then you need to provide IntelliSense with more to work with.
It can also be used by clicking on a word and pressing Ctrl + Space. This is handy when you’ve copied some code that doesn’t quite match your parameters/variables (e.g. Your parameter is “ProductID” but the copied code is “productId”).
Note: Ctrl + Space can also be used to bring up Intellisense